PayChangu Popup provides a simple and convenient payment flow for web. It can be integrated in five easy steps, making it the easiest way to start accepting payments.
An example
Here's what an implementation of PayChangu Inline Popup on a checkout page could look like:
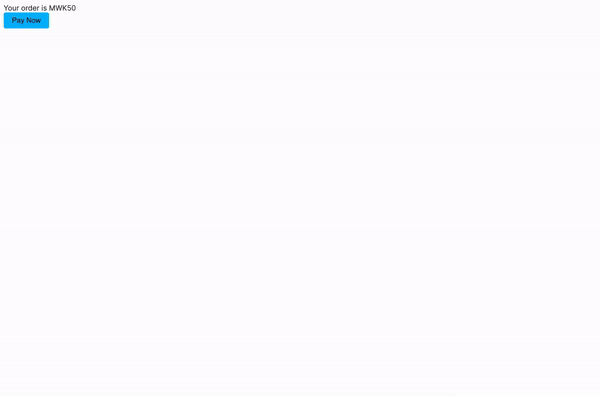
Image code https://codepen.io/paychangu/pen/JjqKWdr
First, you include the PayChangu Inline library with a script tag:
<script src="https://in.paychangu.com/js/popup.js"></script>
Next up is the payment button. This is the button the customer clicks after they've reviewed their order and are ready to pay you. You'll attach an onclick event handler to this button that calls makePayment(), a custom JS function you're going to write.
<div id="wrapper"></div>
<button type="button" onClick="makePayment()">Pay Now</button>
Finally, in the makePayment() function, you call the PayChanguCheckout() function with some custom parameters:
function makePayment(){
PaychanguCheckout({
"public_key": "pub-test-HYSBQpa5K91mmXMHrjhkmC6mAjObPJ2u",
"tx_ref": '' + Math.floor((Math.random() * 1000000000) + 1),
"amount": 1000,
"currency": "MWK",
"callback_url": "https://example.com/callbackurl"",
"return_url": "https://example.com/returnurl",
"customer":{
"email": "[email protected]",
"first_name":"Mac",
"last_name":"Phiri",
},
"customization": {
"title": "Test Payment",
"description": "Payment Description",
},
"meta": {
"uuid": "uuid",
"response": "Response"
}
});
}
</script>
Sample inline Implementation
You can embed PayChangu on your page using our PayChanguCheckout() JavaScript function. The function responds to your request in accordance with your request configurations. If you specify a callback_url in your request, the function will redirect your users to the provided callback URL when they complete the payment.
<form>
<script src="https://in.paychangu.com/js/popup.js"></script>
<div id="wrapper"></div>
<button type="button" onClick="makePayment()">Pay Now</button>
</form>
<script>
function makePayment(){
PaychanguCheckout({
"public_key": "pub-test-HYSBQpa5K91mmXMHrjhkmC6mAjObPJ2u",
"tx_ref": '' + Math.floor((Math.random() * 1000000000) + 1),
"amount": 1000,
"currency": "MWK",
"callback_url": "https://example.com/callbackurl"",
"return_url": "https://example.com/returnurl",
"customer":{
"email": "[email protected]",
"first_name":"Mac",
"last_name":"Phiri",
},
"customization": {
"title": "Test Payment",
"description": "Payment Description",
},
"meta": {
"uuid": "uuid",
"response": "Response"
}
});
}
</script>
After the Payment
Four things will happen when a payment is successful:
- We’ll redirect you to your
callback_url
with statustx_ref
after payment is complete. - We’ll send you a webhook if you have it enabled. Learn more about webhooks and see examples here.
- We’ll send an email receipt to your customer if the payment was successful (unless you’ve disabled this feature).
- We’ll send you an email notification (unless you’ve disabled this feature).
On your server, you should handle the redirect and always verify the final state of the transaction.
What if the Payment Fails?
If the payment attempt fails (for instance, due to insufficient funds), you don’t need to take any action. The payment page will remain open, allowing the customer to try again until the payment succeeds or they choose to cancel. Once the customer cancels or after multiple failed attempts, we will redirect to the redirect_url
with the query parameters tx_ref
and status of failed.
If you have webhooks enabled, we’ll send you a notification for each failed payment attempt. This can be useful if you want to reach out to customers who experienced issues with their payment. See our webhooks guide for an example.